100세까지 코딩
[자바 공부] 제네릭 본문
제네릭(generic)이란?
데이터의 타입을 일반화(generalize)하는 것을 의미한다.
클래스나 메소드에서 사용할 내부 데이터 타입을 외부에서 사용자에 의해 미리 지정한다.
장점
1. 잘못된 타입이 들어오는 것을 컴파일 단계에서 방지할 수 있다.
2. 사용자가 타입을 미리 지정하기 때문에 타입을 체크하고 변환해 줄 필요가 없다 = 관리 편리.
3. 코드의 재사용성이 높아진다.
Ex
- 제네릭 클래스를 사용하지 않으면?
public class IntStack{
final int size;
int[] items;
int top;
public void Push(int item) {}
public int Pop(){}
}
public class FloatStack{
final int size;
float[] items;
int top;
public void Push(float item) {}
public float Pop(){}
}
- 제네릭 클래스를 사용하면?
public class Stack<T>{
final int size;
T[] items;
int top;
public void Push(T item) {}
public T Pop(){}
}
이처럼 구조나 기능이 동일하고 자료형만 차이나는 것을 제네릭을 사용해 하나의 클래스로 정의 가능하다.
제너릭 스택 클래스
class Stack<T>{
final int size;
T[] items;
int top;
public Stack() {this(10);}
public Stack(int s) {
size = s > 0 ? s : 10;
items = (T []) new Object[s];
top = -1;
}
public void Push(T item) {
if(top == size -1) throw new FullException();
items[++top] = item;
}
public T Pop() {
if(top == -1) throw new EmptyException();
return items[top--];
}
}
class FullException extends RuntimeException {
public FullException(){
System.out.println("Stack overflow");
}
}
class EmptyException extends RuntimeException {
public EmptyException(){
System.out.println("Stack is empty");
}
}
public class Main{
public static void main(String[] args) {
Stack<Integer> stack = new Stack<Integer>();
stack.Push(123);
int x = stack.Pop();
System.out.println(x);
stack.Pop();
}
}
결과 :
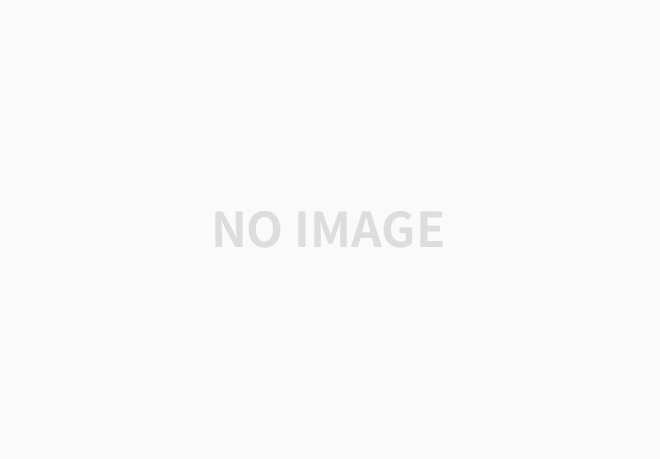
타입 매개변수로는 클래스나 인터페이스만 사용 가능. Stack <Integer>
컬렉션 공부 전 제너릭 알아두기
'JAVA' 카테고리의 다른 글
[자바 공부] 컬렉션(2) Set (0) | 2023.09.12 |
---|---|
[자바 공부] 컬렉션(1) Iterator (0) | 2023.09.12 |
[자바 공부] String 메서드 정리 (0) | 2023.09.08 |
[자바 공부] Arrays 메서드 정리 (0) | 2023.09.08 |
[자바 공부] 형 변환 함수 차이 (parseXXX, valueOf, toString) (0) | 2023.09.04 |